This tutorial details the construction process for a remotely controlled solenoid irrigation valve. In other words, a home computer controls the water flow of an outdoor hose spigot, or bib. The materials cost is about $30-40, excluding the Raspberry Pi (RPi). Cheaper parts can be found with patience and creativity.
The design is intended as a simple introduction to building a complete, personalized home irrigation system. It is also intended to encourage simple DIY solutions to everyday problems. Make modifications and upgrades to suit your needs, resources, and skill level. To conserve water, include drip irrigation and a soil moisture sensor.
Note: This project involves high voltage which requires extreme caution. Always check power connections before touching exposed wires.
Materials
This tutorial assumes the RPi has all GPIO libraries. To install outdoors, the RPi also needs a WiFi adapter and to be accessible by SSH or other remote login.
-- Solenoid Irrigation Valve
This tutorial uses a 24 VAC solenoid for a 3/4" hose spigot.
Some background: there are two main types of solenoids: AC or DC.
- An AC solenoid valve turns water on when voltage is applied, and turns it off when the power is off. The drawback is that it uses AC voltage, requiring an adapter to convert the wall voltage, 120 VAC, into the 24 VAC voltage needed to trigger the valve. Outdoor Installation likely requires an extension cord.
- A DC solenoid valve allows for a battery powered system. It can easily be modified to be wireless and powered by renewable energy using a medium solar panel (~10 W). However, most DC irrigation valves are latching solenoids and require switching the valve lead polarity to turn water on and off.
-- Solid State Relay
The Solid State Relay, or relay, is the intermediary switch between the RPi and the solenoid valve. This tutorial uses a Crouzet Model OAC5-315; its input is 3 - 8 VDC and its output is between 24 - 120 VAC at 1A.
-- N3904 Transistor
-- 4.7 kOhm Resistor
-- PCB Board
Sized to fit the relay, GPIO pins, transistor and resistor.
-- AC Power Adapter (120 VAC to 24 VAC)
Use an extension cord and/or longer leads to install outdoors.
-- 22-gauge stranded wire (insulated), min. 10 feet
-- Waterproof container
I used a leftover project box wrapped with waterproof tape. Cheap/free containers are easy to find; Talenti ice cream containers are an example, and also happen to contain delicious ice cream. With small containers, be sure exposed AC connections are completely covered in epoxy to protect the RPi.
-- Optional: Waterproof connectors, waterproofing tape/lots of duct tape

-- Soldering Iron
-- Wire Strippers
-- Epoxy
Check that it is safe for outdoor use. Marine-grade epoxy may be best for long-term outdoor installation.
-- Screwdriver
-- Optional (but highly recommended): Multimeter
-- Depending on your system container, a drill
Build It!
Hardware Intro: Solenoid Setup
1. Add wire leads to the AC power adapter (if there are none); use at least 3-4 ft of wire.
This AC power adapter has screw-type connectors. Recommended to coat these in epoxy.
2. Verify that the solenoid works by connecting the leads to the power adapter.
The valve makes a "clicking" sound when it is turned on.
For thorough testing, repeat with the valve connected to the hose spigot.
3. Optional: Extend solenoid valve leads using the waterproof connectors.
Twist wires together inside the connectors, check the connection (aka continuity), then epoxy the openings.
Remember, never touch exposed wires when power is on. Always double-check power connections.
If the schematic makes sense, skip the next three hardware steps (Hardware Pts 1 - 3).
Pay attention to the layout of the PCB pads and use them to make connections simpler and more direct. Plan where components are connected prior to soldering. It may be easier to solder components in a different order.
1. Solder the relay to the PCB board.
The labels on the relay tell you the function of each pin. Here's the datasheet for further reference.
1.a. Solder a wire lead to each relay pin, leaving 6 in. or more for the AC leads.

2. Solder the RPi GPIO pin 18, 3.3 VDC pin, and ground pin to PCB board pads.
3. Solder the transistor to the PCB board, keeping each of the legs electrically insulated.
4. Solder one end of the resistor to the middle transistor leg (base pin) and the other end to GPIO pin 18.
Any other available GPIO pin works as long as you change the code to correspond to your chosen pin.
For best results, use one 4.7 kOhm resistor and connect as shown in the photo to the left.
1. Connect the RPi ground pin to transistor pin 1, or emitter pin.
Connect from the flat side of the transistor with a wire, the PCB pads, or a combination. For stranded wire, it helps to twist the ends before pushing them through the PCB holes.
2. Connect transistor pin 3, or collector pin, to the negative DC relay pin.
3. Connect the RPi 3.3 VDC pin to the positive DC relay pin.

Twist wires together and coat in solder. AC current alternates directions, so either lead will work for both the valve and AC power source.

2. Connect the remaining valve lead to one of the relay AC output pins.
3. Connect the remaining AC power source lead to the other relay AC output pin.
4. Check all electrical connections with a multimeter.
If available, check continuity. Otherwise, plug in the AC power source and check that there is ~ 24 VAC across the relay AC pins.
A friendly reminder: Never touch exposed AC connections when the power source is plugged in.
5. Coat all exposed AC connections in epoxy, including the relay AC pins.
For safety purposes and to adhere connections.
1. With that basic principle in mind, here's a simple code to get you started:
2. Run the code in the terminal window of the RPi using the following:
3. Run the program before connecting the AC power source.
Use a multimeter to check that the voltage across the DC relay pins fluctuates from ~ 0VDC to ~ 3.3 VDC in ten second intervals.
4. Plug in the AC power source and run the program again. Listen for the solenoid to click on and off.
Waterproofing
1. Double and triple-check all your connections with a multimeter.
2. Coat remaining exposed connections in epoxy
Give yourself a way to remove the RPi + GPIO cable from the rest of the circuit so the RPi can be used for future projects (if so desired).
3. Place the RPi and PCB board components in a waterproof container.
Find a way to seal the external power cables. The first prototype uses waterproof tape to cushion wires and seal the box. Drilling holes in the box and sealing with epoxy is another quick and easy option.. get creative!
4. Optional: To organize loose wires, twist insulated wires around each other, use zip ties or innovate another method.

Test & Improve!
That's it! Rewrite the program to water your garden as needed. The easiest way is to keep the program as a timer. Change the program to increase the watering time to suit your plant needs and the wait time to >12 hours (>43,200 s).
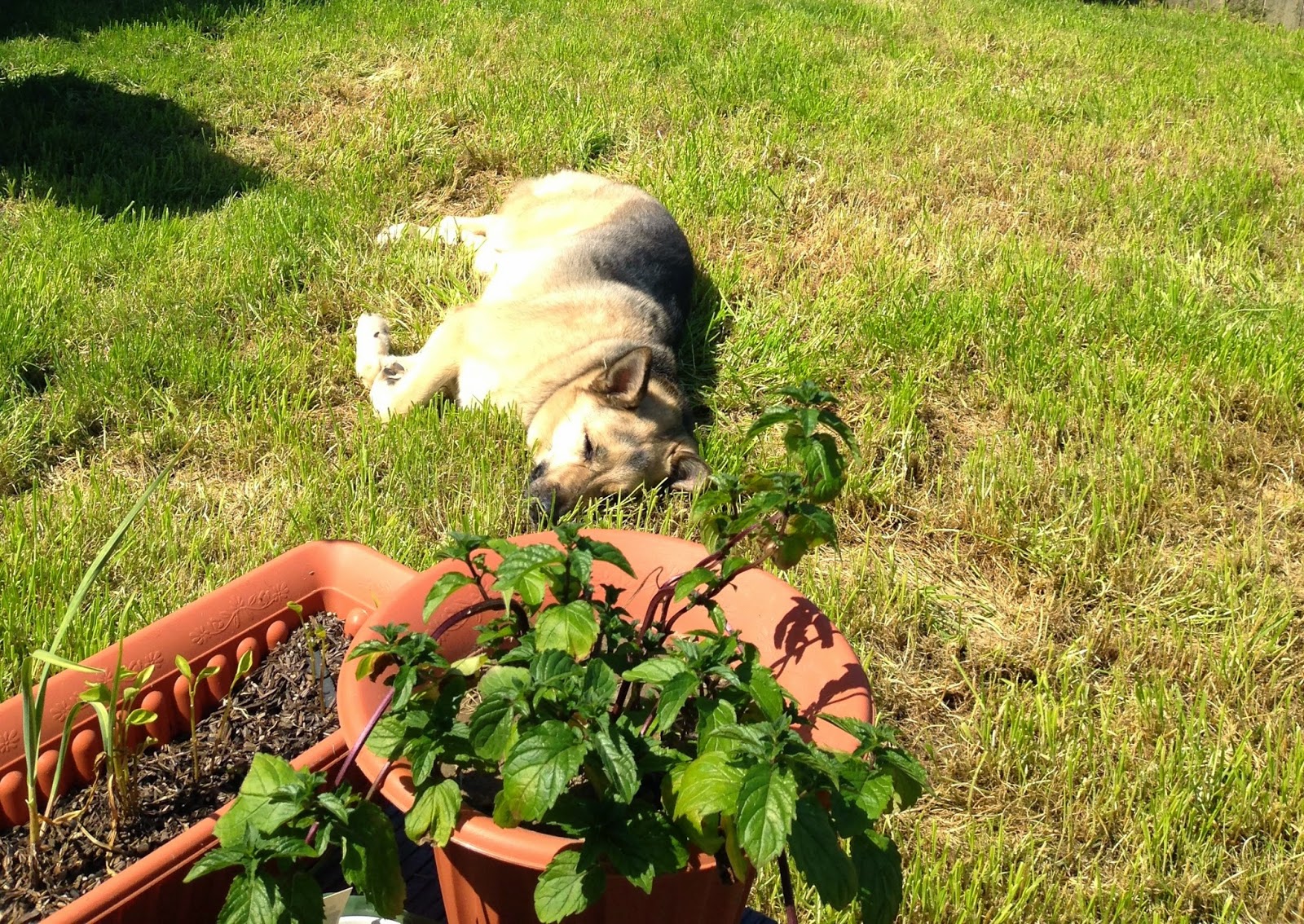
This system was originally designed to be controlled by a RPi-powered soil moisture sensor. To combine the two projects, copy the valve function into the soil moisture sensor program. Update the valve function to turn on if the soil moisture reading is below a certain threshold. Follow the hardware setup as outlined in the soil moisture sensor tutorial. Connect components to the existing PCB board if there is enough space, otherwise get another PCB board for the soil moisture sensing circuit.
Now that you understand the fundamentals, customize and upgrade the system to suit your own needs! Possible extensions include monitoring and/or controlling the system with your phone, or using renewable energy technology for power (e.g. photovoltaics + battery).
This AC power adapter has screw-type connectors. Recommended to coat these in epoxy.
2. Verify that the solenoid works by connecting the leads to the power adapter.
The valve makes a "clicking" sound when it is turned on.
For thorough testing, repeat with the valve connected to the hose spigot.

Twist wires together inside the connectors, check the connection (aka continuity), then epoxy the openings.
Remember, never touch exposed wires when power is on. Always double-check power connections.
Hardware Pt. 1
If the schematic makes sense, skip the next three hardware steps (Hardware Pts 1 - 3).

1. Solder the relay to the PCB board.
The labels on the relay tell you the function of each pin. Here's the datasheet for further reference.
1.a. Solder a wire lead to each relay pin, leaving 6 in. or more for the AC leads.

2. Solder the RPi GPIO pin 18, 3.3 VDC pin, and ground pin to PCB board pads.
3. Solder the transistor to the PCB board, keeping each of the legs electrically insulated.
4. Solder one end of the resistor to the middle transistor leg (base pin) and the other end to GPIO pin 18.
Any other available GPIO pin works as long as you change the code to correspond to your chosen pin.
For best results, use one 4.7 kOhm resistor and connect as shown in the photo to the left.
Hardware Pt. 2
1. Connect the RPi ground pin to transistor pin 1, or emitter pin. Connect from the flat side of the transistor with a wire, the PCB pads, or a combination. For stranded wire, it helps to twist the ends before pushing them through the PCB holes.
2. Connect transistor pin 3, or collector pin, to the negative DC relay pin.
3. Connect the RPi 3.3 VDC pin to the positive DC relay pin.

Hardware Pt. 3
1. Connect one valve lead to one AC power source lead.Twist wires together and coat in solder. AC current alternates directions, so either lead will work for both the valve and AC power source.

2. Connect the remaining valve lead to one of the relay AC output pins.
3. Connect the remaining AC power source lead to the other relay AC output pin.
4. Check all electrical connections with a multimeter.
If available, check continuity. Otherwise, plug in the AC power source and check that there is ~ 24 VAC across the relay AC pins.
A friendly reminder: Never touch exposed AC connections when the power source is plugged in.
5. Coat all exposed AC connections in epoxy, including the relay AC pins.
For safety purposes and to adhere connections.
Software
The software program turns the valve on and off by applying a voltage across the DC terminals of the relay.1. With that basic principle in mind, here's a simple code to get you started:
#Import the necessary libraries
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
#Setup pin 18 as an output
GPIO.setmode(GPIO.BCM)
GPIO.setup(18, GPIO.OUT)
#This function turns the valve on and off in 10 sec. intervals.
def valve_OnOff(Pin):
while True:
GPIO.output(18, GPIO.HIGH)
print("GPIO HIGH (on), valve should be off")
time.sleep(10) #waiting time in seconds
GPIO.output(18, GPIO.LOW)
print("GPIO LOW (off), valve should be on")
time.sleep(10)
valve_OnOff(18)
GPIO.cleanup()

sudo python FileName.py
3. Run the program before connecting the AC power source.
Use a multimeter to check that the voltage across the DC relay pins fluctuates from ~ 0VDC to ~ 3.3 VDC in ten second intervals.
4. Plug in the AC power source and run the program again. Listen for the solenoid to click on and off.
Waterproofing
1. Double and triple-check all your connections with a multimeter.
2. Coat remaining exposed connections in epoxy
Give yourself a way to remove the RPi + GPIO cable from the rest of the circuit so the RPi can be used for future projects (if so desired).

Find a way to seal the external power cables. The first prototype uses waterproof tape to cushion wires and seal the box. Drilling holes in the box and sealing with epoxy is another quick and easy option.. get creative!
4. Optional: To organize loose wires, twist insulated wires around each other, use zip ties or innovate another method.

Test & Improve!
That's it! Rewrite the program to water your garden as needed. The easiest way is to keep the program as a timer. Change the program to increase the watering time to suit your plant needs and the wait time to >12 hours (>43,200 s).
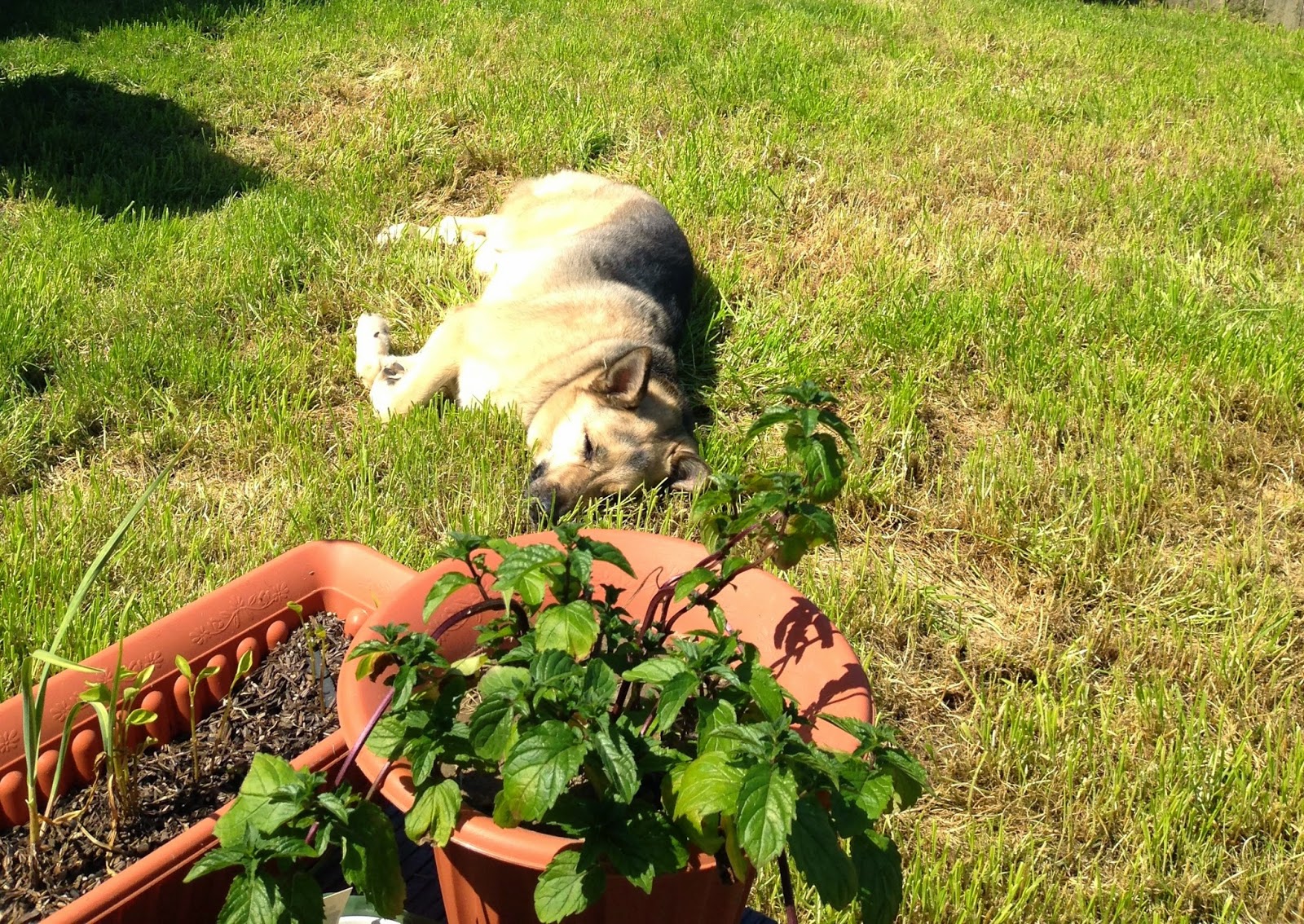
This system was originally designed to be controlled by a RPi-powered soil moisture sensor. To combine the two projects, copy the valve function into the soil moisture sensor program. Update the valve function to turn on if the soil moisture reading is below a certain threshold. Follow the hardware setup as outlined in the soil moisture sensor tutorial. Connect components to the existing PCB board if there is enough space, otherwise get another PCB board for the soil moisture sensing circuit.
Now that you understand the fundamentals, customize and upgrade the system to suit your own needs! Possible extensions include monitoring and/or controlling the system with your phone, or using renewable energy technology for power (e.g. photovoltaics + battery).
Good One!!! Will use this setup for watering my terrace garden
ReplyDeleteThank you! Glad you found it useful!
DeleteThis comment has been removed by a blog administrator.
ReplyDeleteHi,
ReplyDeleteThanks for the great details, i have bookmarked your blog for the future references.
We leading manufacturers of Syntho Glass, Solenoid Valve, Pumps,
Blowers, Expansion Bellows, etc.
This comment has been removed by the author.
ReplyDeleteThanks a lot...very useful to me.
ReplyDeleteGlad that you found it helpful! :D
DeleteHi Jenny...im a student and theres this project that my partner and i need to do that is related to this sprinkler tutorial of yours...i was hoping if you could help us out....
ReplyDeleteHi Sheenay! Sounds like a cool school project! What questions do you have?
DeleteGreat build! I just recently completed a rainwater harvesting system below my deck. I am working on a controller to accomplish the following:
ReplyDelete1) Following a rain event, open the "purge leg". This is where all of the dirty water from the roof and gutters goes first, accumulates then spills over to the tanks.
2) Use a float sensor to "prove" water in the tanks.
3) Operate a re-purposed circ pump to pump water out to the garden beds
4) Measure soil moisture levels and set "desired" moisture level based upon crop/plant. Tomatoes tend to split if water unevenly.
5) Operate control valves to meet the required level.
I will keep you updated as I go, thank you!
Thanks Jen, after searching for many hours for information on a similar system, your write-up is a great find.
ReplyDeleteHello Jenny, I am coaching a team of students for FLL Robotics. Our team decided to do water conservation project for Agricultural and Home gardening purposes. While searching for possibility of soil moisture sensors feeding into the irrigation systems we came across your youtube video. We have few questions. Would you be able to help us?
ReplyDeleteNice explanation of product with the help of images. Very nice.
ReplyDeleteThank you!
dust collector solenoid valve
Retic controller replacement Pretty good post. I just stumbled upon your blog and wanted to say that I have really enjoyed reading your blog posts. Any way I'll be subscribing to your feed and I hope you post again soon. Big thanks for the useful info.
ReplyDelete